UPDATE: A new version of the Complete React on Rails Course is open for pre-orders. Get $10 Off Today.
The webpacker gem has given us an easy way to use JavaScript libraries, including React, inside Rails while using native JS tools like yarn and Webpack.
If you’re using Rails 5.1 or higher, you can directly create a new app with React support out of the box simply by running:
$ rails new myapp --webpack=react
If you’re using an older version of Rails (4.2+), you can install the webpacker gem and then run
$ ./bin/rails webpacker:install $ ./bin/rails webpacker:install:react
Then you simply put your React components in the newly created app/javascript/packs directory and use them with the javascript_pack_tag helper method in a view.
So far, so good.
But if you’ve previously used the react-rails or react_on_rails gem, one thing you might be wondering about is how to get your Rails model data into a React component with webpacker.
Both react-rails and react_on_rails provide a helper method called react_component which you can use in a view to render a component and pass data to it as props.
If we look under the hood, the react_component method puts the Rails data inside a data attribute of a div on the page:
For example, in this calendar appointments app from my Free React on Rails course, we pass appointments data to a component called Appointments with the react_component method:
react_component(‘Appointments’, appointments: @appointments)
If you inspect the source code of the page, you can see the data loaded inside a div with the data attributes react-class and react-props:
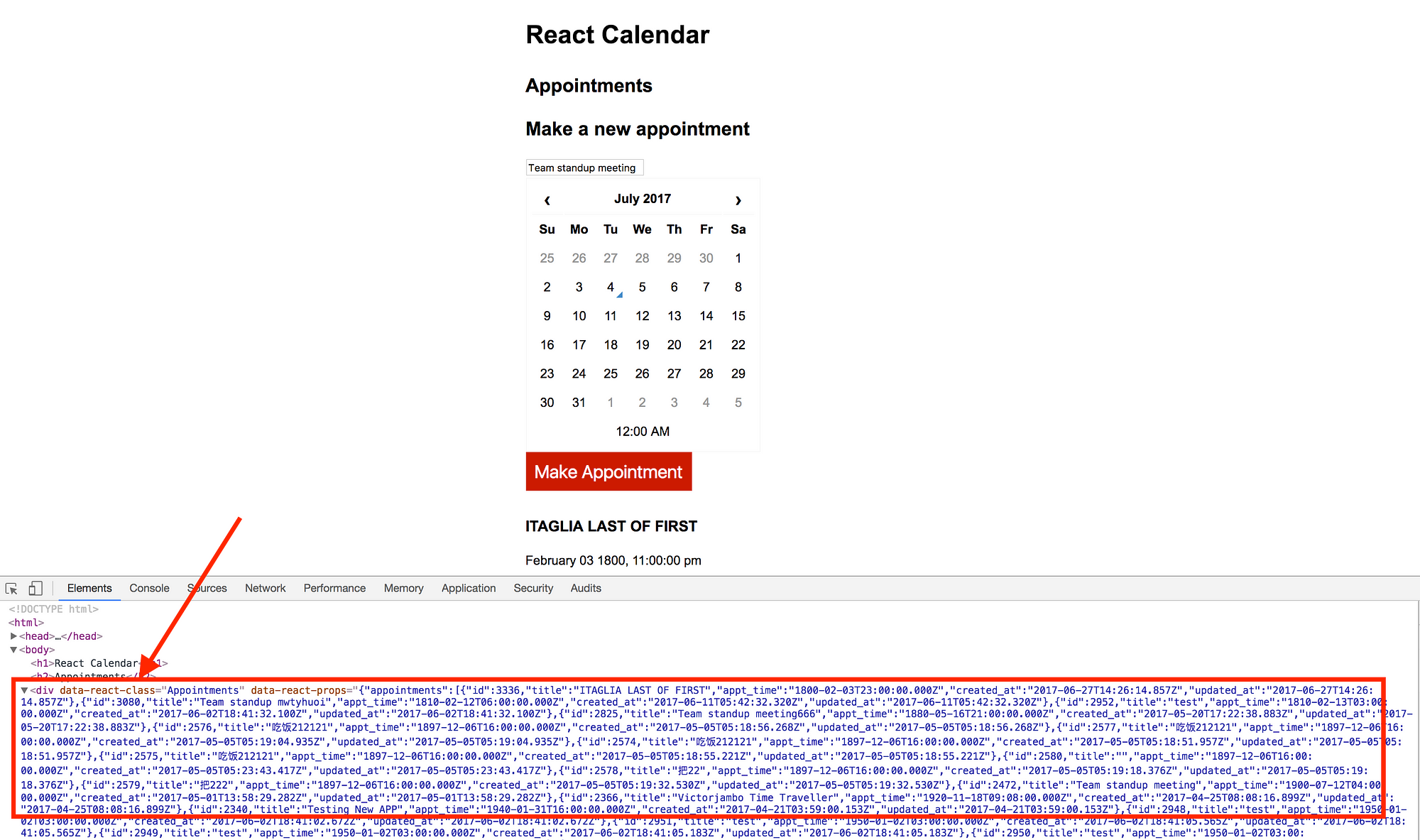
Then, on page load, some JavaScript code (react_ujs) is used to parse the data and pass it on to the React component:
webpacker doesn’t provide any such convenience methods by default.
So we have to write our own code to do the same.
First let’s put the data as JSON inside the data attribute of a div in the view where we want to render the React component:
First let’s put the data as JSON inside the data attribute of a div in the view where we want to render the React component:
<%= content_tag :div, id: "appointments_data", data: @appointments.to_json do %> <% end %>
If you use HAML, you can simply write:
#appointments_data{data: @appointments.to_json}
Then parse the data once all DOM content is loaded and pass it on to the Appointments component:
document.addEventListener('DOMContentLoaded', () => { const node = document.getElementById('appointments_data') const data = JSON.parse(node.getAttribute('data')) ReactDOM.render( <Appointments appointments={data} />, document.body.appendChild(document.createElement('div')), ) })
And that’s all! Your data will now be passed on to the React component.
If you’d rather not do this, you can also use the webpacker-react gem which provides a react_component helper method.
UPDATE: A new version of the Complete React on Rails Course is open for pre-orders. Get $10 Off Today.